How to (Safely) Make A PHP Redirect
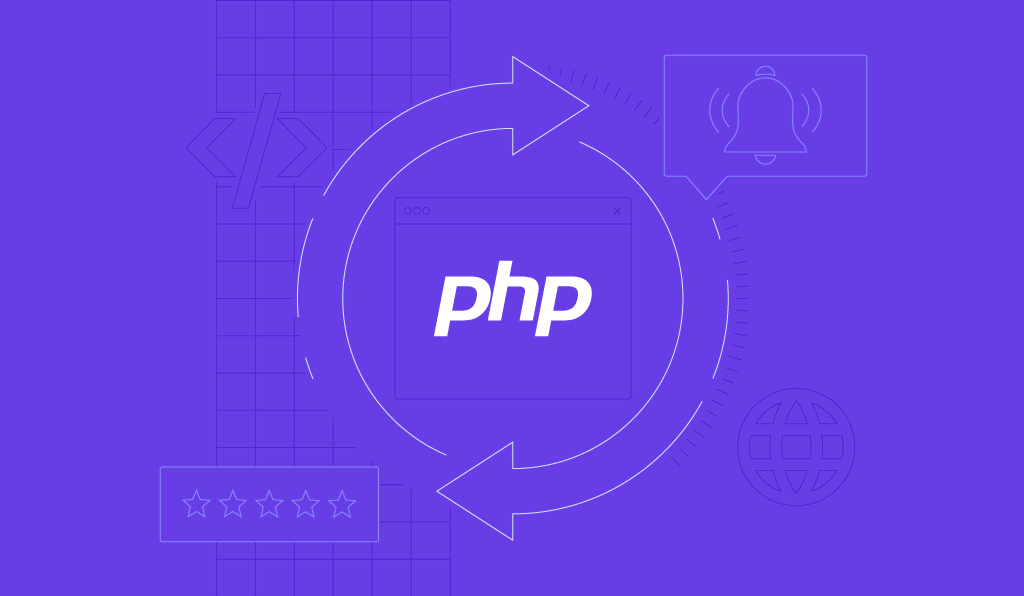
PHP redirects are an incredibly useful tool, but they can also be dangerous if not implemented correctly.
If you’ve been through our introduction to PHP 7.4, and our guide on how to build a website in 5 minutes, you’ll be aware that the header() function can be used to easily redirect a user to another page. In reality, though, using this function is not as simple as it seems. In this guide, we’ll show you how to make a PHP redirect that doesn’t cause big problems further down the line.
The Basic Method for a PHP Redirect
Most guides will tell you that to make a PHP redirect you can just use the header() function at the top of your pages. To do that, you use the function to send a new URL, like this:
header('Location: '.$newURL.php);
This header function should be put before you pass any HTML or text to your users’ browsers, so it should be right at the top of the page. That means it should come before the <!DOCTYPE> declaration, before any Java, and before any PHP code. It will then send users to the new URL.
While it might seem simple, when it comes to the header() function, the simplicity of the code can lead developers into a false sense of security. So let’s look at how you can use this function correctly.
Die() and Exit ()
First, you should use the die() or exit() modifier every time you use a redirect. In summary, the problem is that crawlers and bots are able to ignore headers, and so the page you thought you were redirecting away from is totally accessible to them. If, in other words, you are using a header redirect to protect a particular page, it offers you no protection at all.
That’s why you have to stop processing the rest of the page, in case the redirection is ignored. The way to do that is to append die() or exit() after your redirect:
header("Location: .$newURL.php"); die();
Relative and Absolute URLs
Next, let’s talk about relative and absolute URLs in redirects. RFC 7231 allows you to use both, but you should be extremely careful when using relative redirects. That’s because some website builders collate and rename PHP pages. This means that if you are working on your PHP through a website builder, you may end up breaking all of your redirects.
Unfortunately, at the moment there is no real way around this problem, short of keeping a careful overview of where your redirects are pointing.
Status Codes
The third problem with standard PHP redirects is that PHP’s “location” operator still returns the HTTP 302 code. You should not allow it to do that, because many web browsers implement this code in a way that is totally at odds with the way that it is supposed to function: they essentially use the GET command instead of performing a “real” redirect.
The best practice when building PHP redirects is therefore to specify the code that is returned. Unfortunately, the correct code to use is a point of contention. HTTP 301 indicates a permanent redirect, which might cause you problems with restoring your original page. HTTP 303 is unfortunately understood as “other” by many browsers and can cause problems with indexing your page through search engines.
In practice, and until this situation is resolved, use HTTP 303.
Check The Documentation
Beyond taking the basic precautions above, you should take some time to read the documentation on using PHP redirects before you publish them. You should check the PHP manual to make sure that you understand what you are doing, as well as checking the W3C documentation to ensure that you are following best practice.
And whilst you are catching up on your reading, make sure to also protect a website from common vulnerabilities: if you are already in the position of having to use PHP redirects, it’s likely that your site’s security will need an audit.
Other Methods
Given all these issues, you are probably wondering why you would use a PHP redirect at all. That’s a legitimate question. Though PHP redirects are typically executed more quickly than other types of redirect, and can, therefore, be an important tool in improving website speed, there are other options available.
There are two main approaches to doing this. You can either use the HTML <meta> element to redirect from within the HTML portion of your page, or use JavaScript. The first approach – using <meta> would look like this:
<meta http-equiv="refresh" content="0;url=newpage.php">
The second approach – using JavaScript – is a little more elegant and certainly looks more professional:
window.location.replace("http://newpage.php/");
Both of these approaches will execute a little slower than an immediate header() redirect, but are arguably more flexible.
A Final Word
While following the steps above should mean that your PHP redirects execute securely, if you are in the position of using multiple PHP redirects, it is probably time to rethink the structure of your site.
There are a couple of good reasons for doing that. The first is that not all web hosts are created equal, and if you are sending all of your visitors on a circuitous route around your site, this is going to affect its performance. This can be improved to some degree by using an affordable web hosting provider, but only to a certain extent.
The second reason is that the page you are redirecting from could be collecting data on your visitors without you being aware of it, particularly if you are using web analytics software to track the performance of your site. In our post-GDPR world, that could have significant consequences.
So, in summary: be careful with PHP redirects, use them properly, and only use them where and when you absolutely have to.