What’s New in PHP 8.2: New Features, Deprecations, and Bug Fixes
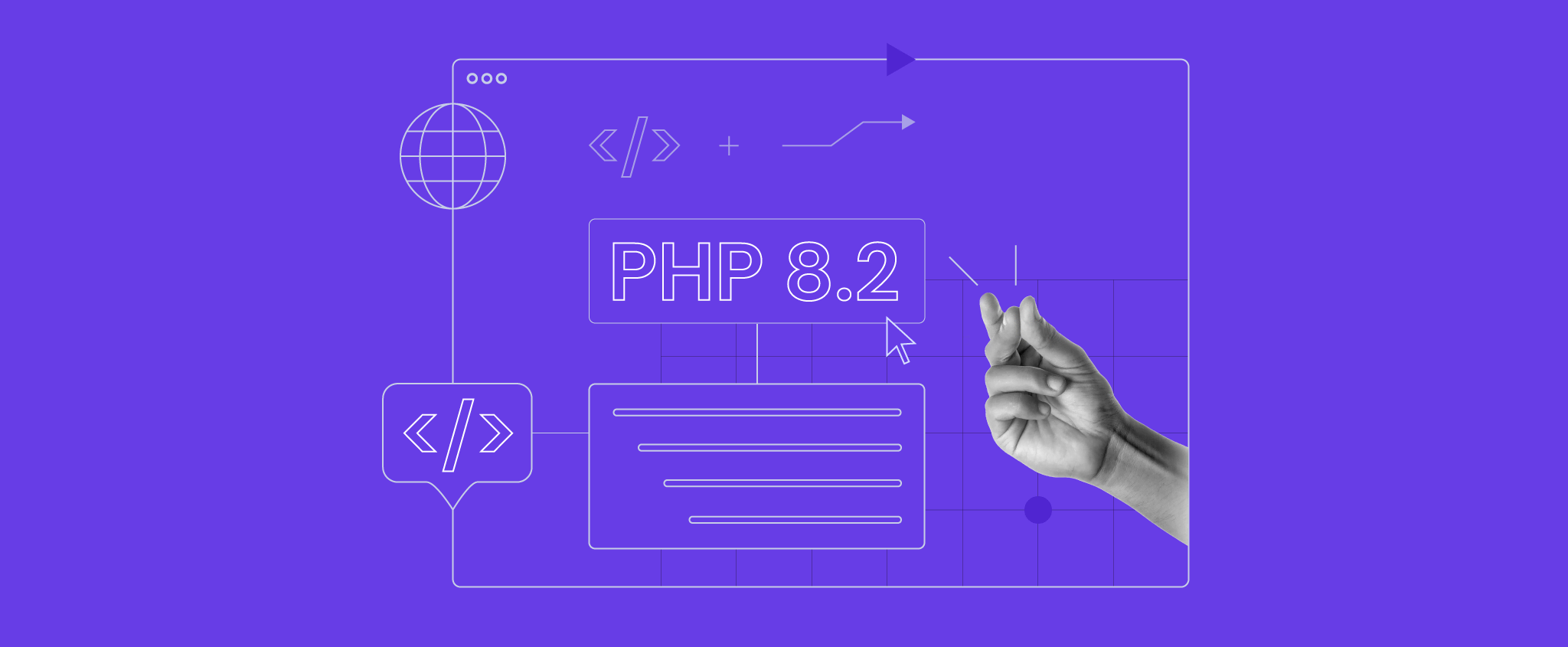
PHP 8.2 is just around the corner. It’s planned to launch on December 8th.
PHP 8.2 is purely aimed at making developers’ life easier. Most of the changes simplify the coding process and deprecate some of the older functions. It’s always recommended to upgrade to the latest PHP version to maximize your site’s security and get used to the new syntax.
Let’s look at all the changes that PHP 8.2 is bringing to the table so you can decide if it’s worth making the switch when the new version eventually comes out.
New PHP 8.2 Features
In this section, we’ll go over the changes and new features introduced with PHP 8.2.
New readonly Classes
The new readonly class property was released with version 8.1. PHP 8.2 improves on it further. Now, you’ll be able to declare a whole class as readonly. Doing so will change all that class’s properties to readonly. This won’t work for dynamic properties – declaring them as readonly will result in an error.
Declaring the class used to look like this:
class ReadOnlyClass { public readonly int $number, public readonly int $anotherNumber }
With PHP 8.2, the process has been thoroughly simplified:
class ReadOnlyClass { public int $number, public int $anotherNumber }
Keep in mind that it won’t be possible to declare the following PHP features:
- Enums – since they cannot contain properties at all.
- Traits.
- Interfaces.
Allow true, null, and false as Standalone Types
With PHP 8.0, users were presented with support for Union Types. You could declare a type as a union of two or more types. Even though you could use false and null as possible types, using them as standalone types was not allowed.
With PHP 8.2, it will be possible to use false and null as standalone types. With this addition, the PHP type system will be more descriptive as you’ll be able to more accurately declare return, parameter, and property types.
Redact Sensitive Parameter Value Support
PHP allows users to track the call stack at any point of the program. It’s extra helpful if you want to debug an application and see why it failed. However, some stack traces contain sensitive information that you may want to mask.
PHP 8.2 will add a new attribute called SensitiveParameter. It prevents sensitive information from being shown or logged whenever an application runs into trouble. In practice, it will look like this:
function passwords( $publicpassword, #[\SensitiveParameter] $secretpassword ) { throw new \Exception('Error'); } passwords('publicpassword', 'secretpassword');
New mysqli_execute_query Function and mysqli::execute_query Method
PHP 8.2 introduces an easier way to handle parameterized MySQLi queries. With the mysqli_execute_query($sql, $params) function and the mysqli::execute_query method, you can prepare, bound, and execute queries within the same function. After successfully running a query, you will be presented with the mysqli_result object.
RFC’s proposed function looks like this:
foreach ($db->execute_query('SELECT * FROM user WHERE name LIKE ? AND type_id IN (?, ?)', [$name, $type1, $type2]) as $row) { print_r($row); }
Allow Constants in Traits
With PHP 8.2, you will be able to declare constants in traits. Until now, traits allowed users to reuse code by defining methods and properties. Now it will be possible to declare constants in traits as well.
Here’s an official RFC proposal example:
trait Foo { public const FLAG_1 = 1; protected const FLAG_2 = 2; private const FLAG_3 = 2; public function doFoo(int $flags): void { if ($flags & self::FLAG_1) { echo 'Got flag 1'; } if ($flags & self::FLAG_2) { echo 'Got flag 2'; } if ($flags & self::FLAG_3) { echo 'Got flag 3'; } } }
New Disjunctive Normal Form (DNF) Types
With PHP 8.2, you will be able to use a new Disjunctive Normal Form (DNF) types feature. It is a standardized way of organizing boolean expressions. To be exact, it consists of a disjunction of conjunctions or simply boolean OR of ANDs.
An RFC proposal example can be found below:
// Accepts an object that implements both A and B, // OR an object that implements D. (A&B)|D // Accepts an object that implements C, // OR a child of X that also implements D, // OR null. C|(X&D)|null // Accepts an object that implements all three of A, B, and D, // OR an int, // OR null. (A&B&D)|int|null
AllowDynamicProperties Attribute
PHP 8.2 will deprecate dynamic variables in classes. This will result in a deprecation message in PHP 8.2 and ErrorException in future versions of PHP.
For this reason, a new #[AllowDynamicProperties] attribute will be added to PHP 8.2 in order to allow dynamic properties for classes. An RFC example looks like this:
class Foo {} $foo = new Foo; // Deprecated: Creation of dynamic property Foo::$bar is deprecated $foo->bar = 1; // No deprecation warning: Dynamic property already exists. $foo->bar = 2;
Deprecated Features in PHP 8.2
In this section, we’ll look at all the features that will be deprecated in PHP 8.2.
#utf8_encode() and utf8_decode() Functions
utf8_encode() and utf8_decode()functions are used to convert between ISO-8859-1 and UTF-8 encoding standards. Due to a lack of error messages, warnings, and limited encoding support, PHP 8.2 will deprecate these functions while PHP 9.0 will exclude them entirely. Alternatively, users will be able to use iconv or intl extensions to convert the encoding standard.
Mbstring: Base64, Uuencode, QPrint, and HTML Entity Encodings
Mbstring is used to convert to and from several character encoding standards such as UTF-8/16/32 and ISO-8859-1. It also includes support for Base64, Quoted-Printable, Uuencode, and HTML Entities.
However, these formats process information in raw bytes instead of sequences of bytes. It’s also worth noting that PHP already has separate functions to encode/decode these formats. Thus, PHP 8.2 will deprecate the mbstring extension with the following labeled encodings:
- BASE64
- UUENCODE
- HTML-ENTITIES
- html (alias of HTML-ENTITIES)
- Quoted-Printable
- qprint (alias of Quoted-Printable)
Partially-Supported Callables
PHP 8.2 will deprecate partially-supported callables that don’t work with the $callable() pattern. The list of deprecated callables can be found below:
$callable = "self::method"; $callable = "parent::method"; $callable = "static::method"; $callable = ["self", "method"]; $callable = ["parent", "method"]; $callable = ["static", "method"]; $callable = ["MyClass", "MyParentClass::myMethod"]; $callable = [new MyClass(), "MyOtherClass::myMethod"];
To prevent the deprecation message, users can convert all self, parent, and static keywords to their corresponding class names.
${var} String Interpolation
PHP allows users to replace variable values within a string literal with double quotes, as in the following examples:
- “$myname” – directly embedding variables.
- “{$myname}” – braces outside the variable.
- “${myname}” – braces after the dollar sign.
- ” ${expr}” – variable variables equivalent to using (string) ${expr}
While offering the same functionality, the syntax of the last two options is quite complex. That’s why they will be deprecated in PHP 8.2. You’ll still be able to use the first two options without any issues.
Other PHP 8.2 Changes
Random Extension Improvement
PHP offers a number of functions for random number generation. With PHP 8.2, some of them will be moved to a new random extension. This extension will be included in PHP by default, and there won’t be an option to disable it.
Here’s a list of all the functions and constants that will be moved to the random extension. Keep in mind that they will remain in the global namespace.
- random_bytes function
- random_int function
- rand function
- getrandmax function
- srand function
- lcg_value function
- mt_rand function
- mt_getrandmax function
- mt_srand function
- MT_RAND_PHP constant
- MT_RAND_MT19937 constant
MySQLi No Longer Supports libmysql
Historically PHP supported two libraries to connect MySQL databases: mysqlnd and libmysql. Since PHP 5.4, the default recommended library has been mysqlnd. In order to simplify PHP code testing, it was decided to remove libmysql with PHP 8.2.
From now on, you’ll need to use the mysqlnd library to connect MySQL databases.
Sort Order Changes for the ksort Function
The ksort function sorts an array by key in ascending order. PHP 8.2 will introduce a bug fix to make the output of the SORT_REGULAR parameter consistent with the other parameters. Up until PHP 8.2, it prioritized alphabetic keys before numeric keys.
Now, ksort will place numeric keys before alphabetic keys when sorting. In practice, sorted keys will look like this:
["1" => '', "2" => '', "a" => '', , "b" => ''];
Instead of:
["a" => '', "b" => '', "1" => '', , "2" => ''];
The str_split Function Returns Empty Arrays for Empty Strings
Another bug fix is meant for the str_split function. It is used to split a string into an array.
Up until PHP 8.2, if this function was given an empty string, it would also return an empty string. PHP 8.2 introduces a fix to this bug, and str_split will return an empty array if given an empty string.
How to Change the PHP Version
Usually, hosting providers offer you an easy way to switch between PHP versions. If you’re using Hostinger’s hPanel, all you need to do is select your hosting plan and search for PHP Configuration:
Here, you will find all of the latest stable versions of PHP. Select the preferred version and press Update to save the changes.
Within this utility, It’s also possible to manage PHP extensions and PHP options for all versions individually.
Conclusion
PHP 8.2 offers many improvements over the previous versions. It introduces a number of new features like new readonly classes, deprecates several outdated implementations with complex syntax, and fixes important bugs to streamline the development workflow and make using PHP easier.
We hope this post has helped you prepare for the upcoming PHP 8.2 launch. We’re eagerly looking forward to the new version’s full release on December 8th!
Comments
March 31 2023
It's still not available in my Cloud hosting, though.
January 11 2023
Thanks for this blog. It's nice to see the changes they've made in just a couple of lines